Kontrole i korekty¶
Niestandardowe automatyczne korekty¶
You can also implement your own automatic fixup in addition to the standard ones and
include them in AUTOFIX_LIST
.
The automatic fixes are powerful, but can also cause damage; be careful when writing one.
For example, the following automatic fixup would replace every occurrence of the string
foo
in a translation with bar
:
# Copyright © Michal Čihař <michal@weblate.org>
#
# SPDX-License-Identifier: GPL-3.0-or-later
from django.utils.translation import gettext_lazy
from weblate.trans.autofixes.base import AutoFix
class ReplaceFooWithBar(AutoFix):
"""Replace foo with bar."""
name = gettext_lazy("Foobar")
def fix_single_target(self, target, source, unit):
if "foo" in target:
return target.replace("foo", "bar"), True
return target, False
To install custom checks, provide a fully-qualified path to the Python class
in the AUTOFIX_LIST
, see Custom quality checks, add-ons and auto-fixes.
Dostosowywanie zachowania za pomocą flag¶
You can fine-tune Weblate’s behavior by using flags. The flags provide visual feedback to the translators and help them to improve their translation. The flags are merged from following sources:
Source string, see Dodatkowe informacje o ciągach źródłowych.
Per-string flags extracted from the file format, see Obsługiwane formaty plików.
Translation flags (currently only
read-only
flag for bilingual source string).File-format specific flags.
The flags are comma-separated; if they have parameters, they are separated with colon. You can use quotes to include whitespaces or special characters in the string. For example:
placeholders:"special:value":"other value", regex:.*
Both single and double quotes are accepted, special characters are being escaped using backslash:
placeholders:"quoted \"string\"":'single \'quoted\''
placeholders:r"^#*"
To verify that translators do not change the heading of a Markdown document: A failing check will be triggered if the string «### Index» is translated as «# Indice»
placeholders:r"\]\([^h].*?\)"
To ensure that internal links are not being translated (i.e. [test](../checks) does not become [test](../chequeos).
Here is a list of flags currently accepted:
rst-text
Treat a text as an reStructuredText document, affects Niezmienione tłumaczenie.
dos-eol
Uses DOS end-of-line markers instead of Unix ones (
\r\n
instead of\n
).read-only
The string is read-only and should not be edited in Weblate, see Ciągi tylko do odczytu.
terminology
Used in Słownik. Copies the string into all glossary languages so it can be used consistently in all translations. Also useful in combination with
read-only
, for example in product names.priority:N
Priority of the string. Higher priority strings are presented first for translation. The default priority is 100, the higher priority a string has, the earlier it is offered for translation.
max-length:N
Limit the maximal length for a string to N characters, see Maksymalna długość tłumaczenia.
xml-text
Treat text as XML document, affects Składnia XML and Znaczniki XML.
font-family:NAME
Define font-family for rendering checks, see Zarządzanie czcionkami.
font-weight:WEIGHT
Define font-weight for rendering checks, see Zarządzanie czcionkami.
font-size:SIZE
Define font-size for rendering checks, see Zarządzanie czcionkami.
font-spacing:SPACING
Define letter spacing for rendering checks, see Zarządzanie czcionkami.
icu-flags:FLAGS
Zdefiniuj flagi do dostosowywania zachowania kontroli jakości ICU MessageFormat.
icu-tag-prefix:PREFIX
Ustaw wymagany prefiks dla tagów XML dla kontroli jakości ICU MessageFormat.
placeholders:NAME:NAME2:...
Placeholder strings expected in translation, see Symbole zastępcze.
replacements:FROM:TO:FROM2:TO2...
Replacements to perform when checking resulting text parameters (for example in Maksymalny rozmiar tłumaczenia or Maksymalna długość tłumaczenia). The typical use case for this is to expand placeables to ensure that the text fits even with long values, for example:
replacements:%s:"John Doe"
.variants:SOURCE
Mark this string as a variant of string with matching source. See Warianty ciągów.
regex:REGEX
Wyrażenie regularne pasujące do tłumaczenia, zobacz: ref: check-regex.
forbidden
Indicates forbidden translation in a glossary, see Zabronione tłumaczenia.
strict-same
Make „Unchanged translation” avoid using built-in words blacklist, see Niezmienione tłumaczenie.
strict-format
Make format checks enforce using format even for plural forms with a single value, see Formatowanie ciągów.
check-glossary
Włącz kontrolę jakości Nie jest zgodny z słownikiem.
angularjs-format
Włącz kontrolę jakości Ciąg znaków interpolacji AngularJS.
c-format
Włącz kontrolę jakości Format C.
c-sharp-format
Włącz kontrolę jakości Format C#.
es-format
Włącz kontrolę jakości Literały szablonu ECMAScript.
i18next-interpolation
Enable the interpolacja i18next quality check.
icu-message-format
Włącz kontrolę jakości ICU MessageFormat.
java-printf-format
Enable the Format Java quality check.
java-format
Włącz kontrolę jakości Format Java Message.
javascript-format
Włącz kontrolę jakości Format JavaScript.
lua-format
Włącz kontrolę jakości Format Lua.
object-pascal-format
Włącz kontrolę jakości Format Object Pascal.
percent-placeholders
Włącz kontrolę jakości Procent symboli zastępczych.
perl-brace-format
Enable the Format nawiasów klamrowych Perla quality check.
perl-format
Włącz kontrolę jakości Format języka Perl.
php-format
Włącz kontrolę jakości Format PHP.
python-brace-format
Enable the Format klamrowy Python quality check.
python-format
Enable the Format Python quality check.
qt-format
Włącz kontrolę jakości Format Qt.
qt-plural-format
Włącz kontrolę jakości Forma liczby mnogiej Qt.
ruby-format
Włącz kontrolę jakości Format Ruby.
scheme-format
Włącz kontrolę jakości Format Scheme.
vue-format
Włącz kontrolę jakości Formatowanie Vue I18n.
md-text
Treat text as a Markdown document, and provide Markdown syntax highlighting on the translation text area. Enables Łącza Markdown, Odniesienia do Markdown, and Składnia Markdown quality checks.
case-insensitive
Adjust checks behavior to be case-insensitive. Currently affects only Symbole zastępcze quality check.
safe-html
Włącz kontrolę jakości Niebezpieczny HTML.
url
The string should consist of only a URL. Enable the URL quality check.
ignore-all-checks
Ignoruj wszystkie kontrole jakości.
ignore-bbcode
Pomiń kontrolę jakości Znaczniki BBCode.
ignore-duplicate
Pomiń kontrolę jakości Kolejne zduplikowane wyrazy.
ignore-check-glossary
Pomiń kontrolę jakości Nie jest zgodny z słownikiem.
ignore-double-space
Pomiń kontrolę jakości Podwójna spacja.
ignore-angularjs-format
Pomiń kontrolę jakości Ciąg znaków interpolacji AngularJS.
ignore-c-format
Pomiń kontrolę jakości Format C.
ignore-c-sharp-format
Pomiń kontrolę jakości Format C#.
ignore-es-format
Pomiń kontrolę jakości Literały szablonu ECMAScript.
ignore-i18next-interpolation
Pomiń kontrolę jakości interpolacja i18next.
ignore-icu-message-format
Pomiń kontrolę jakości ICU MessageFormat.
ignore-java-printf-format
Skip the Format Java quality check.
ignore-java-format
Pomiń kontrolę jakości Format Java Message.
ignore-javascript-format
Pomiń kontrolę jakości Format JavaScript.
ignore-lua-format
Pomiń kontrolę jakości Format Lua.
ignore-object-pascal-format
Pomiń kontrolę jakości Format Object Pascal.
ignore-percent-placeholders
Pomiń kontrolę jakości Procent symboli zastępczych.
ignore-perl-brace-format
Skip the Format nawiasów klamrowych Perla quality check.
ignore-perl-format
Pomiń kontrolę jakości Format języka Perl.
ignore-php-format
Pomiń kontrolę jakości Format PHP.
ignore-python-brace-format
Pomiń kontrolę jakości Format klamrowy Python.
ignore-python-format
Pomiń kontrolę jakości Format Python.
ignore-qt-format
Pomiń kontrolę jakości Format Qt.
ignore-qt-plural-format
Pomiń kontrolę jakości Forma liczby mnogiej Qt.
ignore-ruby-format
Pomiń kontrolę jakości Format Ruby.
ignore-scheme-format
Pomiń kontrolę jakości Format Scheme.
ignore-vue-format
Pomiń kontrolę jakości Formatowanie Vue I18n.
ignore-translated
Pomiń kontrolę jakości Zostało przetłumaczone.
ignore-inconsistent
Pomiń kontrolę jakości Niespójność.
ignore-kashida
Pomiń kontrolę jakości Użyto litery Kashida.
ignore-md-link
Pomiń kontrolę jakości Łącza Markdown.
ignore-md-reflink
Pomiń kontrolę jakości Odniesienia do Markdown.
ignore-md-syntax
Pomiń kontrolę jakości Składnia Markdown.
ignore-max-length
Pomiń kontrolę jakości Maksymalna długość tłumaczenia.
ignore-max-size
Pomiń kontrolę jakości Maksymalny rozmiar tłumaczenia.
ignore-escaped-newline
Pomiń kontrolę jakości Niedopasowane \n.
ignore-end-colon
Pomiń kontrolę jakości Niedopasowany dwukropek.
ignore-end-ellipsis
Pomiń kontrolę jakości Niedopasowane wielokropki.
ignore-end-exclamation
Pomiń kontrolę jakości Niedopasowany wykrzyknik.
ignore-end-stop
Pomiń kontrolę jakości Niedopasowana kropka.
ignore-end-question
Pomiń kontrolę jakości Niedopasowany znak zapytania.
ignore-end-semicolon
Pomiń kontrolę jakości Niedopasowany średnik.
ignore-newline-count
Pomiń kontrolę jakości Niedopasowanie podziałów wierszy.
ignore-plurals
Pomiń kontrolę jakości Brakująca forma liczby mnogiej.
ignore-placeholders
Pomiń kontrolę jakości Symbole zastępcze.
ignore-punctuation-spacing
Pomiń kontrolę jakości Odstępy między czcionkami.
ignore-regex
Pomiń kontrolę jakości Wyrażenie regularne.
ignore-reused
Skip the Ponownie użyte tłumaczenie quality check.
ignore-same-plurals
Pomiń kontrolę jakości Te same liczby mnogie.
ignore-begin-newline
Pomiń kontrolę jakości Początek od nowej linii.
ignore-begin-space
Pomiń kontrolę jakości Spacje początkowe.
ignore-end-newline
Pomiń kontrolę jakości Zakończenie nową linią.
ignore-end-space
Pomiń kontrolę jakości Końcowa spacja.
ignore-same
Pomiń kontrolę jakości Niezmienione tłumaczenie.
ignore-safe-html
Pomiń kontrolę jakości Niebezpieczny HTML.
ignore-url
Pomiń kontrolę jakości URL.
ignore-xml-tags
Pomiń kontrolę jakości Znaczniki XML.
ignore-xml-invalid
Pomiń kontrolę jakości Składnia XML.
ignore-zero-width-space
Pomiń kontrolę jakości Znak spacji o zerowej szerokości.
ignore-ellipsis
Pomiń kontrolę jakości Wielokropek.
ignore-icu-message-format-syntax
Pomiń kontrolę jakości Składnia ICU MessageFormat.
ignore-long-untranslated
Pomiń kontrolę jakości Długo nieprzetłumaczone.
ignore-multiple-failures
Pomiń kontrolę jakości Wiele nieudanych kontroli.
ignore-unnamed-format
Pomiń kontrolę jakości Wiele nienazwanych zmiennych.
ignore-optional-plural
Pomiń kontrolę jakości Niespluralizowane.
Informacja
Generally the rule is named ignore-*
for any check, using its
identifier, so you can use this even for your custom checks.
These flags are understood both in Konfiguracja komponentu settings, per source string settings and in the translation file itself (for example in GNU gettext).
Wymuszanie kontroli¶
You can configure a list of checks which can not be ignored by setting Wymuszone kontrole in Konfiguracja komponentu. Each listed check can not be dismissed in the user interface and any string failing this check is marked as Needs editing (see Stan tłumaczenia).
Informacja
Turning on check enforcing doesn’t enable it automatically. The check can be turned on by adding the corresponding flag to string or component flags.
Zobacz także
Zarządzanie czcionkami¶
Podpowiedź
Fonts uploaded into Weblate are used purely for purposes of the Maksymalny rozmiar tłumaczenia check, they do not have an effect in Weblate user interface.
The Maksymalny rozmiar tłumaczenia check used to calculate dimensions of the rendered text needs font to be loaded into Weblate and selected using a translation flag (see Dostosowywanie zachowania za pomocą flag).
Weblate font management tool in Fonts under the Manage menu of your translation project provides interface to upload and manage fonts. TrueType or OpenType fonts can be uploaded, set up font-groups and use those in the check.
The font-groups allow you to define different fonts for different languages, which is typically needed for non-latin languages:
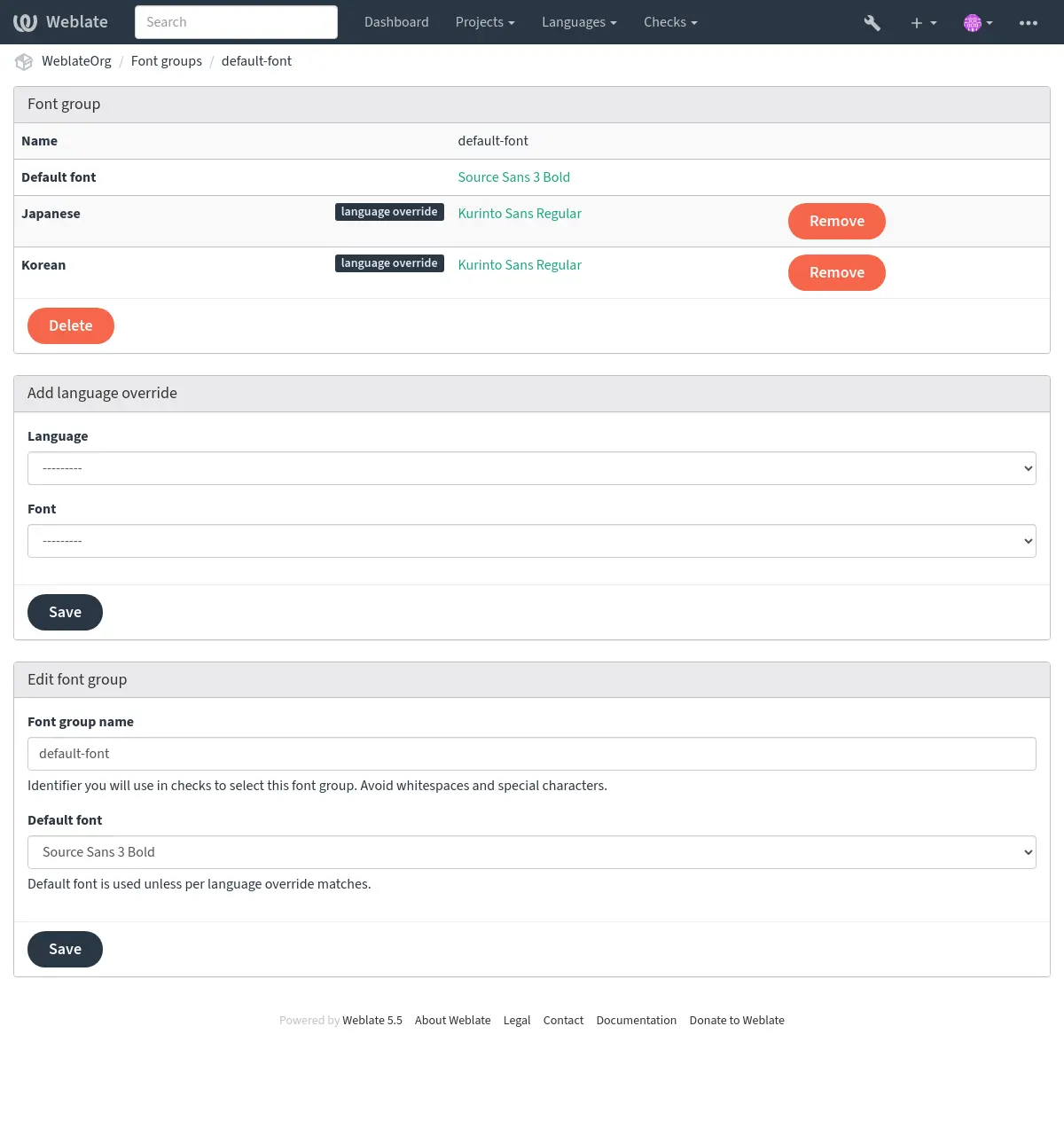
The font-groups are identified by name, which can not contain whitespace or special characters, so that it can be easily used in the check definition:
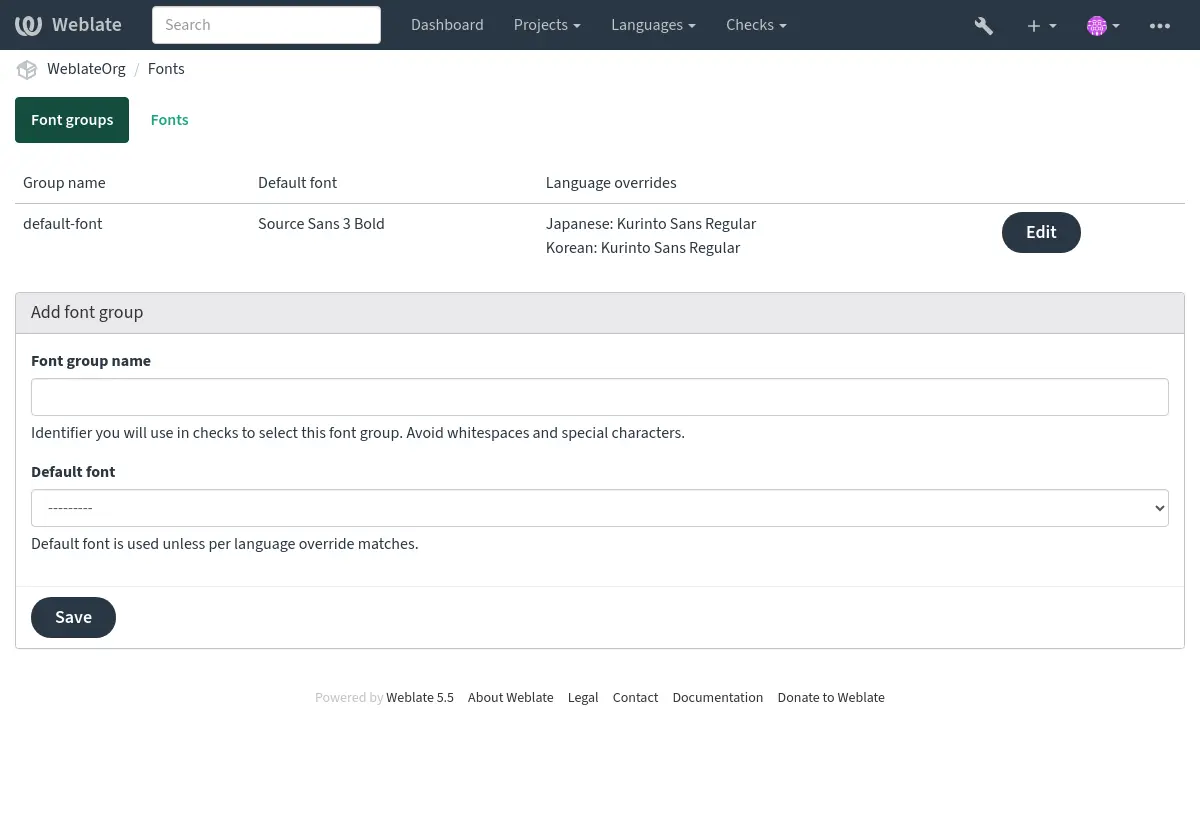
Font-family and style is automatically recognized after uploading them:
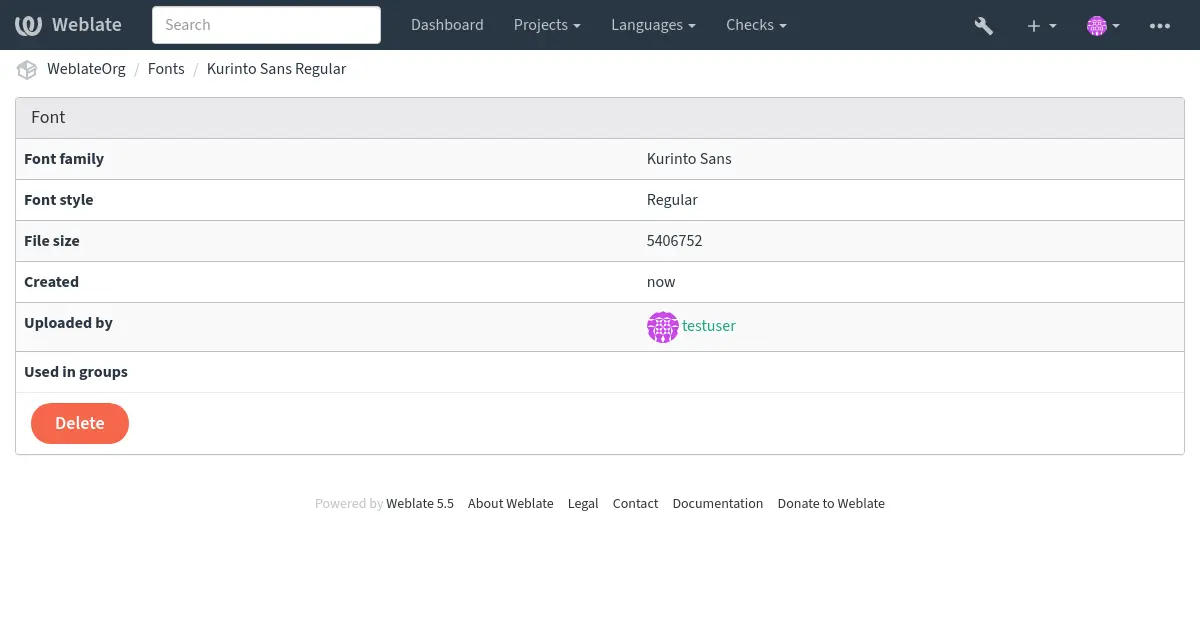
You can have a number of fonts loaded into Weblate:
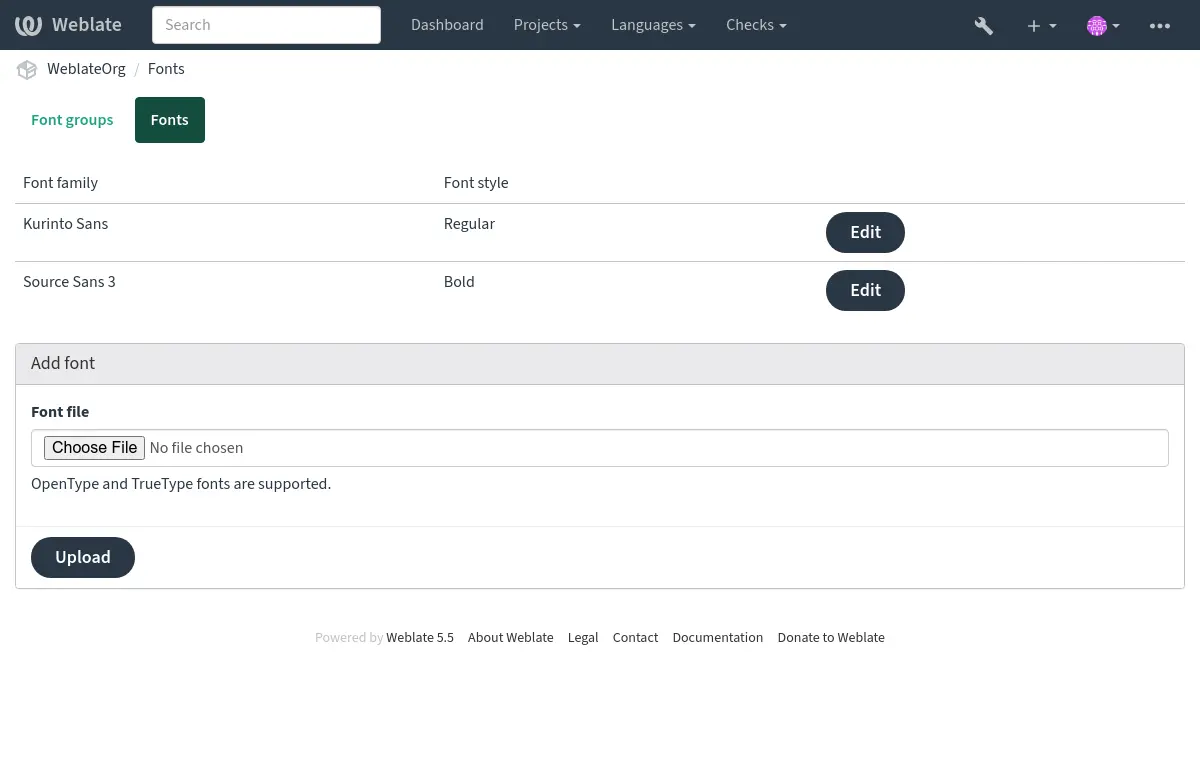
To use the fonts for checking the string length, pass it the appropriate flags (see Dostosowywanie zachowania za pomocą flag). You will probably need the following ones:
max-size:500
/max-size:300:5
Defines maximal width in pixels and, optionally, the maximum number of lines (word wrapping is applied).
font-family:ubuntu
Defines font group to use by specifying its identifier.
font-size:22
Określa rozmiar czcionki w pikselach.
Pisanie własnych kontroli¶
A wide range of quality checks are built-in, (see Kontrole jakości), though
they might not cover everything you want to check. The list of performed checks
can be adjusted using CHECK_LIST
, and you can also add custom checks.
Podklasa weblate.checks.Check
Ustaw kilka atrybutów.
Implement either the
check
(if you want to deal with plurals in your code) or thecheck_single
method (which does it for you).
Kilka przykładów:
To install custom checks, provide a fully-qualified path to the Python class
in the CHECK_LIST
, see Custom quality checks, add-ons and auto-fixes.
Checking translation text does not contain „foo”¶
This is a pretty simple check which just checks whether the translation is missing the string „foo”.
# Copyright © Michal Čihař <michal@weblate.org>
#
# SPDX-License-Identifier: GPL-3.0-or-later
"""Simple quality check example."""
from django.utils.translation import gettext_lazy
from weblate.checks.base import TargetCheck
class FooCheck(TargetCheck):
# Used as identifier for check, should be unique
# Has to be shorter than 50 characters
check_id = "foo"
# Short name used to display failing check
name = gettext_lazy("Foo check")
# Description for failing check
description = gettext_lazy("Your translation is foo")
# Real check code
def check_single(self, source, target, unit):
return "foo" in target
Checking that Czech translation text plurals differ¶
Check using language info to verify the two plural forms in Czech language are not same.
# Copyright © Michal Čihař <michal@weblate.org>
#
# SPDX-License-Identifier: GPL-3.0-or-later
"""Quality check example for Czech plurals."""
from django.utils.translation import gettext_lazy
from weblate.checks.base import TargetCheck
class PluralCzechCheck(TargetCheck):
# Used as identifier for check, should be unique
# Has to be shorter than 50 characters
check_id = "foo"
# Short name used to display failing check
name = gettext_lazy("Foo check")
# Description for failing check
description = gettext_lazy("Your translation is foo")
# Real check code
def check_target_unit(self, sources, targets, unit):
if unit.translation.language.is_base(("cs",)):
return targets[1] == targets[2]
return False
def check_single(self, source, target, unit) -> bool:
"""We don't check target strings here."""
return False